Warning
You are reading the documentation for an older Pickit release (2.4). Documentation for the latest release (3.4) can be found here.
The Pickit URCap interface
Pickit integrates seamlessly with Universal Robots by means of a URCap plugin. This plugin allows to perform vision-guided pick and place with minimal programming effort. For installation instructions please refer to the URCap installation and setup article.
This article documents the global variables exposed by the pick and place template, as well as additional commands and helper functions that can be used in conjuction with it.
Global variables
Picking
These variables are used by the pick and place template in the Pick sequence. They refer to the currently selected object for picking.
PickitPick |
---|
Object pick point, represented as a pose variable. |
PickitPrePick |
---|
Object pre-pick point, represented as a pose variable. This point is used for performing a linear approach motion to PickitPick. The strategy used for computing the pre-pick point can be specified in the Pick node configuration. |
PickitPostPick |
---|
Object post-pick point, represented as a pose variable. This point is used for performing a linear retreat motion from PickitPick. The strategy used for computing the post-pick point can be specified in the Pick node configuration. |
Advanced picking
These variables expose additional information that can be used when picking an object. They refer to the currently selected object for picking, and are not used by default by the pick and place template. Learn more about when and how you should use them in the smart placing examples.
PickitPickId |
---|
ID of the pick point that was selected for picking, represented as an integer. |
PickitPickRefId |
---|
ID of the pick point with respect to which PickitPickId was created, represented as an integer. If the pick point associated to PickitPickId was not created with respect to another pick point, this value will be the same as PickitPickId. |
PickitPickOff |
---|
Pick point offset, represented as a pose variable. This is the relative transformation between the reference pick point (identified by PickitPickRefId) and the pick point that was selected for picking (identified by PickitPickId). If the
|
Object information
These variables expose additional information about the object beyond where to pick it. They refer to the latest object detection results sent by Pickit, and are not used by default by the pick and place template.
PickitObjType |
---|
Object type, represented as an integer. The mapping between the object type and its identifier is the following:
Pickit Flex and Pattern
|
PickitObjDim |
---|
Object dimensions, in meters, represented as a 3D array. Depending on the object type, the array should be interpreted as follows:
Pickit Flex and Pattern
|
PickitObjAge |
---|
Object age, represented as a floating-point number. This object age is the duration, in seconds, elapsed between the capturing of the camera image and the moment the object information is sent to the robot. |
Commands
This section presents a set of commands that add to Polyscope’s existing ones. In Polyscope 5, they can be inserted in a robot program by selecting Program in the header bar, then, on the left panel URCaps → Pickit: commands.
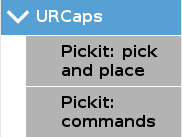
Next, navigate to the Command tab on the right panel select an entry from the Pickit command drop-down.
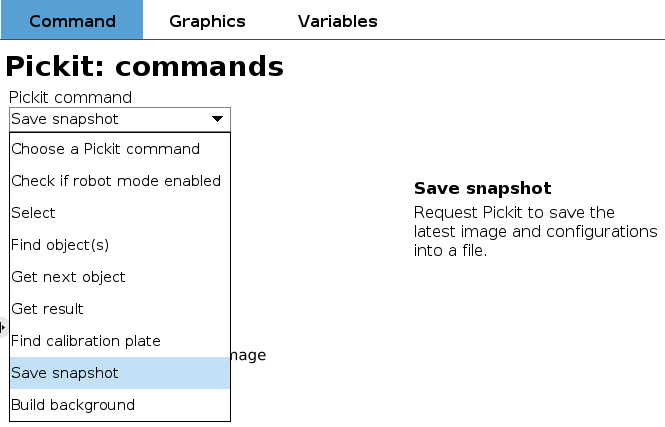
Picking.
These are optional commands that can be added to a pick and place robot program, for instance, inside one of the sequences of the pick and place template.
Save snapshot |
---|
Save a snapshot with the latest detection results. The saved snapshot can then be loaded or downloaded by going to the Snapshots page on the Pickit web interface and searching for a file whose name contains the capture timestamp. Example usage: Trigger saving a snapshot on the action after end sequence, when no objects are found (but the ROI is not empty), so it’s possible to investigate the cause. |
Build background |
---|
Build the background cloud used by some of the advanced Region of Interest filters. Calling this function will trigger a camera capture, so if the camera mount is fixed, the robot must not occlude the camera view volume. If instead the camera is robot-mounted, the robot must be in the detection point (more). |
Calibration
There is a single command meant to be used in a calibration robot program, and not in a pick and place program.
Find calibration plate |
---|
Trigger detection of the robot-camera calibration plate. This command requires the Pickit web interface to be in the Calibration page, hence robot mode should be disabled. When Pickit is not in the Calibration page, a pop-up is shown. |
Helper functions
Helper functions return useful information about picking. They are typically used as the expression of a conditional, such as an if
statement, and can be selected from the Function
drop-down.
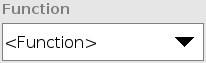
pickit_empty_roi() |
---|
Call this function in the optional action after end sequence to check if there are no more pickable objects because of an empty Region of Interest (ROI). This check is included in the default implementation of action after end. Return |
pickit_no_object_found() |
---|
Call this function in the optional action after end sequence to check if there are no more pickable objects because the requested object is not found, but the ROI is not empty. This check is included in the default implementation of action after end. Return |
pickit_no_object_reachable() |
---|
Call this function in the optional action after end sequence to check if there are no more pickable objects because they are detected but unreachable by the robot. This check is included in the default implementation of action after end. Return |
pickit_no_image_captured() |
---|
Call this function in the optional action after end sequence to check if there are no more pickable objects because Pickit failed to capture a camera image. When this is the case, it typically indicates a hardware disconnection issue, such as a loose connector or broken cable. This function can be used as trigger to send an alarm to a higher level monitoring system. This check is included in the default implementation of action after end. Return |
pickit_remaining_objects() |
---|
Call this function in the Pick, Place, or the optional action after end sequence to query the remaining number of detected (but potentially unpickable) objects from the last object detection run. Return The number of remaining detected objects. |
Low-level interface
Apart from the pick and place template, Pickit offers a low-level interface. It exists mainly for backward compatibility with the URCap version 1, and for power users that need to implement application logic that cannot be represented by the pick and place template.