The Pickit low-level URCap interface
This article documents the Pickit low-level URCap interface, which consists of a set of global variables, commands and helper functions that allow you to explicitly write the logic of a pick and place application. A companion article describes an example robot program written using the low-level interface.
Note
The low-level interface exists mainly for backward compatibility with the URCap version 1, and for power users that need to implement application logic that cannot be represented by the pick and place template.
The pick and place template is the preferred way to set up a new pick and place application, as it requires a lower programming effort, and implements a robust execution logic under the hood.
Global variables
Most of the global variables are exposed by the pick and place template.
Note
The URCap plugin exposes global variables under their version 2 names (CamelCase
), and also under the version 1 names (snake_case
) for backward compatibility.
For new programs, the version 2 names are recommended.
PickitPick, also exposed as
pickit_pose
.PickitPrePick, also exposed as
pickit_pre_pose
.PickitObjType, also exposed as
pickit_type
.PickitObjDim, also exposed as
pickit_dim
.
Detection
These variables are updated upon each detection, and refer to the currently selected object for picking. They are used by the pick and place template in the Pick sequence.
PickitPick |
---|
Object pick point, represented as a pose variable. |
PickitPrePick |
---|
Object pre-pick point, represented as a pose variable. This point is used for performing a linear approach motion to PickitPick. The strategy used for computing the pre-pick point can be specified in the Pick node configuration. |
PickitPostPick |
---|
Object post-pick point, represented as a pose variable. This point is used for performing a linear retreat motion from PickitPick. The strategy used for computing the post-pick point can be specified in the Pick node configuration. |
These variables also refer to the currently selected object for picking, and expose additional information that can be used when picking an object. They are not used by default by the pick and place template. Learn more about when and how you should use them in the smart placing examples.
PickitPickId |
---|
ID of the pick point that was selected for picking, represented as an integer. |
PickitPickRefId |
---|
ID of the pick point with respect to which PickitPickId was created, represented as an integer. If the pick point associated to PickitPickId was not created with respect to another pick point, this value will be the same as PickitPickId. |
PickitPickOff |
---|
Pick point offset, represented as a pose variable. This is the relative transformation between the reference pick point (identified by PickitPickRefId) and the pick point that was selected for picking (identified by PickitPickId). If the
|
These variables expose additional information about the object beyond where to pick it. They also refer to the latest object detection results sent by Pickit, and are not used by default by the pick and place template.
PickitObjType |
---|
Object type, represented as an integer. The mapping between the object type and its identifier is the following:
Pickit Flex and Pattern
|
PickitObjDim |
---|
Object dimensions, in meters, represented as a 3D array. Depending on the object type, the array should be interpreted as follows:
Pickit Flex and Pattern
|
PickitObjAge |
---|
Object age, represented as a floating-point number. This object age is the duration, in seconds, elapsed between the capturing of the camera image and the moment the object information is sent to the robot. |
Calibration
These global variables are updated every time a new robot-camera calibration is performed.
PickitCal |
---|
The calibration transform,
This variable is updated by pickit_compute_calib(). |
PickitCalErr |
---|
Calibration error, represented as a pose variable. This variable is updated by pickit_validate_calib(). |
PickitCalErrPos |
---|
Position calibration error, represented as a floating-point number (in millimeters). This value contains the norm of the calibration error translational component. This variable is updated by pickit_compute_calib() and pickit_validate_calib(). |
PickitCalErrAng |
---|
Orientation calibration error, represented as a floating-point number (in degrees). This value contains the angle of the calibration error rotational component, computed using the axis-angle representation. This variable is updated by pickit_compute_calib() and pickit_validate_calib(). |
Commands
This section presents a set of commands that add to Polyscope’s existing ones. In Polyscope 5, they can be inserted in a robot program by selecting Program in the header bar, then, on the left panel URCaps → Pickit: commands.
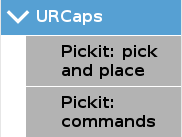
Next, navigate to the Command tab on the right panel and select an entry from the Pickit command drop-down.
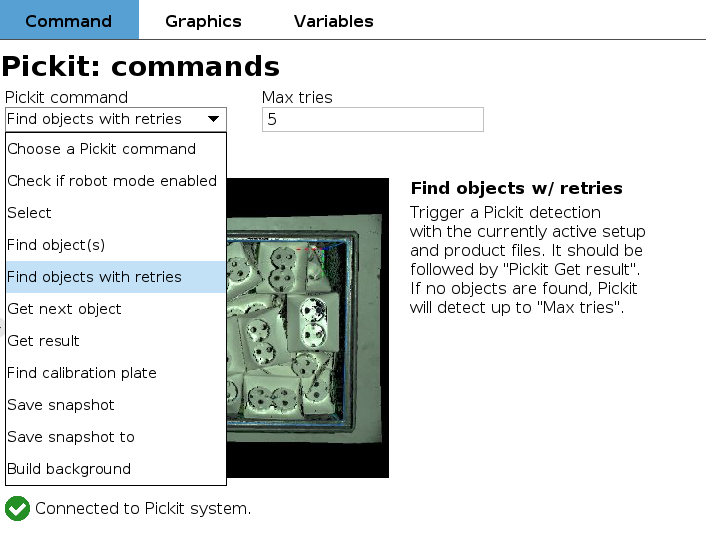
System commmands
The commands in this section are related to the Pickit system’s state.
Check if robot mode enabled |
---|
Checks whether robot mode is enabled in Pickit.
All commands listed below require robot mode to be enabled. |
Detection commands
The following commands take care of triggering detections and collecting the results.
Find object(s) |
---|
Trigger a Pickit object detection using the currently active setup and product configuration. This call should be followed by a call to Get result, which waits until a response for the detection request is ready. Note that it’s valid (and sometimes encouraged) to perform robot motions or other non Pickit actions between calls to Find objects and Get result, for instance. Refer to the cycle time optimization section of the Pick and place program article for the motivation behind performing robot motions while a Pickit detection is ongoing. |
Get next object |
---|
Request the next detected object. A single call to Find object(s) or Find objects with retries might yield the detection of multiple objects. Get next object allows to request the next available object, if any, without the need of triggering a new detection and the time overhead it entails. The next Pickit command after Get next object should always be Get result, which waits until a response for the request is ready. It’s recommended to use this command only when objects in the detection region have not moved (significantly) since calling Find object(s). A good example of when to use Get next object is when a detection is unreachable by the robot. An example of when using Get next object is not ideal would be the following bin picking scenario:
When the objects in the detection region have moved, it’s better to re-trigger Find object(s) or Find objects with retries instead. |
Find objects with retries |
---|
Trigger a Pickit object detection with retries, using the currently active setup and product configuration. This call should be followed by a call to Get result, which waits until a response for the detection request is ready. Parameters
Refer to the Find objects command for usage tips. |
Get result |
---|
Wait for Pickit to reply with detection results.
Get result should always be the next Pickit command after
a Find object(s), Find objects with retries or
Get next object request.
It blocks until a reply from Pickit is received, and the success of the
request can then be queried by calling
Parameters
|
Configuration commands
Select |
---|
Loads the specified setup and product configuration. This configuration specifies the behavior of Pickit detections, e.g. what to look for, in which part of the field of view. Parameters
Available configurations are listed in drop-down menus. Note: If you need to change setup or product based on a runtime decision (not known in advance), consider using the pickit_configure helper instead. |
Build background |
---|
Build the background cloud used by some of the advanced Region of Interest filters. Calling this function will trigger a camera capture, so if the camera mount is fixed, the robot must not occlude the camera view volume. If instead the camera is robot-mounted, the robot must be in the detection point (more). |
Calibration commands
The following command is necessary when performing a robot-camera calibration from the robot.
Find calibration plate |
---|
Trigger a calibration plate detection. If the plate detection fails, the program is terminated. Plate detection failure can occur if the plate is not completely visible, or if the marker detection is poor. |
Monitoring commands
Save snapshot |
---|
Save a snapshot with the latest detection results. The saved snapshot can then be loaded or downloaded by going to the Snapshots page on the Pickit web interface and searching for a file whose name contains the capture timestamp. Example usage: Trigger saving a snapshot on the action after end sequence, when no objects are found (but the ROI is not empty), so it’s possible to investigate the cause. |
Save snapshot to |
---|
Save a snapshot with the latest detection results to a specific subfolder. The saved snapshot can then be loaded or downloaded by going to the Snapshots page on the Pickit web interface and searching for a file whose name contains the capture timestamp. Parameters
|
Scripting functions
In addition to the URCap commands, the Pickit interface also offers URScript functions.
These functions can be selected from the Function
drop-down of the script editor.
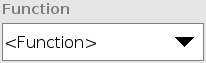
System functions
System functions interact with Pickit at a system level. This function allows the programmer to manually finish pick and place.
pickit_break() |
---|
This function allows the programmer to manually finish pick and place. Call this function from any of the sequences (Object detection, Pick or Place) to finish the program, when an application-specific condition is met. ![]() Pick and place finishes after the current sequence completes.
In the above example, Once the sequence from which See also pickit_break_called(). |
pickit_break_called() |
---|
This function checks if pickit_break() was called. This check is included in the default implementation of action after end. Return |
pickit_shutdown() |
---|
Cleanly shut down the Pickit processor. Return |
Detection functions
pickit_capture_image() |
---|
Capture a camera image without triggering object detection. This function blocks until image capture has completed, and is especially useful when working with a robot-mounted camera, where the robot must remain stationary during image capture, but not during detection. This function is meant to be used prior to calling pickit_process_image(). If the camera moves during image capture, a warning will be shown in the web interface. For fixed camera mounts, it’s usually more convenient to call functions that combine image capture and processing, like Find object(s) or Find objects with retries.
|
pickit_process_image() |
---|
Trigger a Pickit object detection without image capture using the currently active setup and product configuration. This function uses the latest captured camera image, which typically comes from calling pickit_capture_image() just before. This call should be paired with a call to Get result, which waits until a response for he detection request is ready. Refer to the documentation of pickit_capture_image() to learn more about the recommended usage of this function. |
pickit_object_found() |
---|
Check if the last detection found at least one pickable object. When
Return A similar function |
pickit_no_object_found() |
---|
Check if the last detection found no pickable objects because the requested object is not found, but the ROI is not empty. This check is included in the default implementation of action after end of the pick and place template. If you are not using the template, but the low-level URCap commands, you can call this function after a Get result. Return A similar function |
pickit_object_reachable() |
---|
Check if the object retried by the last call to Get result has reachable pick and pre-pick poses.
|
pickit_no_object_reachable() |
---|
Check if all detected and pickable objects are not reachable by the robot. This check is included in the default implementation of action after end. If you are not using the template, but the low-level URCap commands, you can call this function after a Get result. Return |
pickit_no_image_captured() |
---|
Check if object detection was unsuccessful due to a failure to capture a camera image. When this is the case, it typically indicates a hardware disconnection issue, such as a loose connector or broken cable. This function can be used as trigger to send an alarm to a higher level monitoring system. This check is included in the default implementation of action after end. Return |
pickit_empty_roi() |
---|
Check if Pickit detected an empty Region of Interest (ROI) during the last detection. An empty ROI is one of the possible causes for Pickit to find no pickable objects. This function is called in the optional action after end sequence of the pick and place template. If you are not using the template, but the low-level URCap commands, you can call this function after a Get result.
|
pickit_remaining_objects() |
---|
Get the number of remaining object detections. After calling Get result, this function returns the total number of object detections minus one, as the first object data is available through the Global variables. This value is also equal to the number of times Get next object can be called. As such, the returned value decreases with each call to Get next object.
|
Configuration functions
pickit_configure(setup_id, product_id) |
---|
Loads the specified setup and product configuration. This function is similar to the Select command, but specifies the setup and product by their respective IDs (integers) as opposed to names from a drop-down. Prefer using this function over the Select command when you need to change the setup or product based on a runtime decision, and the IDs are read from variable values. Parameters
|
The following are advanced functions that can save and modify the Pickit configuration.
pickit_save_product() |
---|
Save the active product file. Return |
pickit_save_setup() |
---|
Save the active setup file. Return |
pickit_set_cylinder_dim(length, diameter) |
---|
Set the dimensions of a Teach cylinder model (diameter and length). When this call succeeds, the active product file will have unsaved changes. If desired, you can save them by calling pickit_save_product(). Learn more about the conditions that apply to this request here. Parameters
Return |
Calibration functions
pickit_configure_calib() |
---|
Set the robot-camera calibration configuration using the calibration method, camera mount and transform (if applicable) already set in the web interface calibration wizard. Equivalent to calling the advanced version (see below) like so: method = 3 # METHOD_DEFAULT.
camera_mount = 0 # Ignored.
transform = p[0, 0, 0, 0, 0, 0] # Ignored.
pickit_configure_calibration(method, camera_mount, transform)
Return |
pickit_configure_calib(method, camera_mount, transform) |
---|
Set the robot-camera calibration configuration (advanced version). Parameters
Return |
pickit_find_calib_plate() |
---|
Trigger detection of the robot-camera calibration plate. You can alternatively call the Find calibration plate URCap command, which doesn’t return a boolean, but instead terminates program execution when failing to detect the plate. Return |
pickit_compute_calib() |
---|
Trigger the computation of the robot-camera calibration. When the function returns Return |
pickit_validate_calib(distance_tol, angle_tol) |
---|
Validate if the current robot-camera calibration is still valid. It’s considered valid if the location of the detected calibration plate is within the specified distance and angular tolerances. When the function returns Parameters
Return |