KUKA bin picking program
This program requires that Pickit is installed and set up with your robot. For installation instructions, please refer to the KUKA installation and setup article.
Attention
The examples contained in the Pickit application files contain hard-coded robot poses that should be adapted to every new robot. When executing such programs for the first time, please do so in manual mode and at low speed to check for potential collisions.
The PickitBinPicking program
This program can be found in R1 > Program > Pickit.
Note
This program only works with Pickit software version of 2.2 or greater. If you are using a software version prior 2.2, please contact us at support@pickit3d.com, and we will assist you in finding a solution.
DEF PickitBinPicking( )
;FOLD Collision detection settings
;---------------------------------
;Collision recovery for KSS 8.5 and 8.6
;Set the correct LOAD DATA
;Declare the collision detection interrupt to stop the search motion
INTERRUPT DECL 25 WHEN $CYCFLAG[255] == TRUE DO RetreatWhenFound()
$CYCFLAG[255]=FALSE
;Define a cycle flag on the peak values for all axes
$CYCFLAG[255]=(($COLLMON_MAX[1]>iSearchSensitivity[1]) OR($COLLMON_MAX[2]>iSearchSensitivity[2])OR($COLLMON_MAX[3]>iSearchSensitivity[3])OR($COLLMON_MAX[4]>iSearchSensitivity[4])OR($COLLMON_MAX[5]>iSearchSensitivity[5])OR($COLLMON_MAX[6]>iSearchSensitivity[6]))
;Start collision monitoring in the system
;The data set ColDetect[1] is here used as the active data set
;for global collision detection
;Use ColDetect[1] for all motions of the robot except those in the pick()
;routine where the collision recovery is used
;ColDetect[1] can be edited in Configuration->Collision Detection
USE_CD_PARAMS(1)
;ENDFOLD
PTP XHOME
IF NOT Pickit_is_running() THEN
PickitMessages(#info, 1)
HALT
ENDIF
;Fill in correct setup and product id
Pickit_configure(1,1)
;Set the tool used for picking, make sure the TCP is defined correctly
BAS(#TOOL,1)
BAS(#BASE,0)
PTP XDetect
WAIT SEC 0.0 ;Wait until the robot reaches the position
Pickit_detect_with_retr(5)
WAIT FOR Pickit_get_results()
LOOP ; ---- Main Loop
IF Pickit_object_found() THEN
;Five-points extraction path
F_Pick=Pickit_get_pose()
;F_RelFrame is used to apply the prepick and postpick offsets
F_RelFrame={X 0,Y 0,Z 0,A 0,B 0,C 0}
F_RelFrame.Z=PrePickOffset
F_PrePick=F_RelFrame:F_Pick
F_RelFrame.Z=PostPickOffset
F_PostPick=F_RelFrame:F_Pick
F_BinEntry=F_PrePick
F_BinExit=F_PostPick
F_BinEntry.Z=BinEntryZ
F_BinExit.Z=BinExitZ
;Check if positions are reachable
IF IsReachable() THEN
PTP XAbovePickitArea
;FOLD set collision recovery parameters for the picking sequence
;Activate the collisions detection interrupt
INTERRUPT ON 25
;Reset the peak values
RESET_CM_MAX()
;Make sure the cycle flag is low before starting the motion
WAIT FOR NOT $CYCFLAG[255]
;ENDFOLD
;Picking sequence
;This is the search routine where collision recovery will be applied
Pick()
;FOLD reset collision recovery parameters after picking is done
;Go back to the global collision detection settings
;If a collision happens now, the robot will stop
USE_CD_PARAMS(1)
;Deactivate the collisions detection interrupt
INTERRUPT OFF 25
;Make sure the advance run is not leaving the pick() subroutine
;before the interrupt is deactivated
WAIT SEC 0
;ENDFOLD
PTP XAbovePickitArea
PTP XDetect
WAIT SEC 0.0
Pickit_capture_image()
Pickit_process_image()
;Drop the picked part at the needed locationS
PTP XDrop
;------- Add release logic
WAIT FOR Pickit_get_results()
IF NOT Pickit_object_found() THEN
;Retry detecting if no object found during the first detection.
PTP XDetect
WAIT SEC 0.0
Pickit_detect_with_retr(4)
WAIT FOR Pickit_get_results()
ENDIF
ELSE ;Position unreachable, try picking the next object
PickitMessages(#info,2)
Pickit_next_object()
WAIT FOR Pickit_get_results()
ENDIF
ELSE ;No Object found, exit
IF Pickit_roi_empty() THEN
PickitMessages(#info, 3)
EXIT
ELSE
PickitMessages(#info, 4)
Pickit_save_scene()
EXIT
ENDIF
ENDIF
ENDLOOP
END
The structure of this program is similar to the simple pick and place program.
Program subroutines
In this program, the picking motion commands are executed in a separate subroutine. To pick a detected part, the robot needs to follow the five point extraction path recommended for picking in deep bins. More information about the five-point extraction path can be found in the robot-independent complete pick and place program.
DEF Pick()
;Conservative LIN motion parameters inside the bin
BAS(#VEL_CP,30)
BAS(#ACC_CP,20)
LIN F_BinEntry
LIN F_PrePick
LIN F_Pick
;----- Add grasping logic
WAIT SEC 1.0 ;Wait until grasping is completed
LIN F_PostPick
LIN F_BinExit
END
The IsReachable()
subroutine is defined to perform a reachability check on the five points of the extraction path:
DEFFCT BOOL IsReachable()
Pickit_ErrStatCheckPos1=0
Pickit_ErrStatCheckPos2=0
Pickit_ErrStatCheckPos3=0
Pickit_ErrStatCheckPos4=0
Pickit_ErrStatCheckPos5=0
$TARGET_STATUS=#SOURCE
;Note: The INVERSE function cannot guarantee that a LIN motion will be feasible
PickitAxistest=INVERSE(F_Pick,xAbovePickitArea,Pickit_ErrStatCheckPos1)
PickitAxistest=INVERSE(F_PrePick,xAbovePickitArea,Pickit_ErrStatCheckPos2)
PickitAxistest=INVERSE(F_PostPick,xAbovePickitArea,Pickit_ErrStatCheckPos3)
PickitAxistest=INVERSE(F_BinEntry,xAbovePickitArea,Pickit_ErrStatCheckPos4)
PickitAxistest=INVERSE(F_BinExit,xAbovePickitArea,Pickit_ErrStatCheckPos5)
IF (Pickit_ErrStatCheckPos1 == 0) AND (Pickit_ErrStatCheckPos2 == 0) AND (Pickit_ErrStatCheckPos3 == 0) AND (Pickit_ErrStatCheckPos4 == 0) AND (Pickit_ErrStatCheckPos5 == 0) THEN
RETURN(TRUE)
ELSE
MsgNotify("Pose Unreachable by the Robot")
RETURN(FALSE)
ENDIF
ENDFCT
The RetreatWhenFound()
subroutine is called when a collision is detected inside the Pick()
subroutine.
It performs a recovery motion to F_PostPick
before resuming the PickitBinPicking()
routine.
DEF RetreatWhenFound()
;Deactivate the collision detection interrupt
INTERRUPT OFF 25
;Stop the active robot motion
BRAKE FF
;FOLD Message: Give peak value of collided axes
IF ($COLLMON_MAX[6]>iSearchSensitivity[6])THEN
MsgNotify( "Measured peak A6 = %1","Collision recov", $COLLMON_MAX[6])
MsgNotify( "Collision limit A6 = %1","Collision recov", iSearchSensitivity[6])
ENDIF
IF ($COLLMON_MAX[5]>iSearchSensitivity[5])THEN
MsgNotify( "Measured peak A5 = %1","Collision recov", $COLLMON_MAX[5])
MsgNotify( "Collision limit A5 = %1","Collision recov", iSearchSensitivity[5])
ENDIF
IF ($COLLMON_MAX[4]>iSearchSensitivity[4])THEN
MsgNotify( "Measured peak A4 = %1","Collision recov", $COLLMON_MAX[4])
MsgNotify( "Collision limit A4 = %1","Collision recov", iSearchSensitivity[4])
ENDIF
IF ($COLLMON_MAX[3]>iSearchSensitivity[3])THEN
MsgNotify( "Measured peak A3 = %1","Collision recov", $COLLMON_MAX[3])
MsgNotify( "Collision limit A3 = %1","Collision recov", iSearchSensitivity[3])
ENDIF
IF ($COLLMON_MAX[2]>iSearchSensitivity[2])THEN
MsgNotify( "Measured peak A2 = %1","Collision recov", $COLLMON_MAX[2])
MsgNotify( "Collision limit A2 = %1","Collision recov", iSearchSensitivity[2])
ENDIF
IF ($COLLMON_MAX[1]>iSearchSensitivity[1])THEN
MsgNotify( "Measured peak A1 = %1","Collision recov", $COLLMON_MAX[1])
MsgNotify( "Collision limit A1 = %1","Collision recov", iSearchSensitivity[1])
ENDIF
WAIT SEC 2
;ENDFOLD
;-------- Add release logic in case a part was picked
;To recover from the collision, skip to the last pose in the picking sequence
;This is important because the tool could still be inside the bin
LIN F_PostPick
WAIT SEC 0
;Reactivate the collision detection interrupt
INTERRUPT ON 25
;Reset the peak values
RESET_CM_MAX()
;Make sure the cycle flag is low before starting the motion
WAIT FOR NOT $CYCFLAG[255]
;Add this command if you want to resume the PickitSimplePicking() routine
RESUME
END
Note
Depending on which Pickit software version you are running, the program can look different, even though the functionality is the same. We recommend to use the program shipped with your Kuka connect version
Define the tool for picking
Create a tool with the actual TCP values. Make sure that the tool has the correct load data. In this program, #TOOL1 is used.
Set correct input arguments
The commands Pickit_configure
and Pickit_detect_with_retr
need input arguments.
See Pickit communication functions for more information about these arguments.
Define fixed points
In this program, 4 fixed points are used. These points need to be defined depending on the application.
Home: Where the robot will start the program.
Detect: Where to perform object detection from.
AbovePickArea: A point roughly above the pick area from which the above two can be reached without collision.
Drop: Where to place objects.
Pick sequence settings
The five-point extraction path can be modified with these four variables defined in the respective .DAT
file:
PrePickOffset: the z-offset for
F_PrePick
relative to the tool frame.PostPickOffset: the z-offset for
F_PostPick
relative to the tool frame.binEntryZ: the z absolute value for
F_BinEntry
relative to the robot base.binExitZ: the z absolute value for
F_BinExit
relative to the robot base.
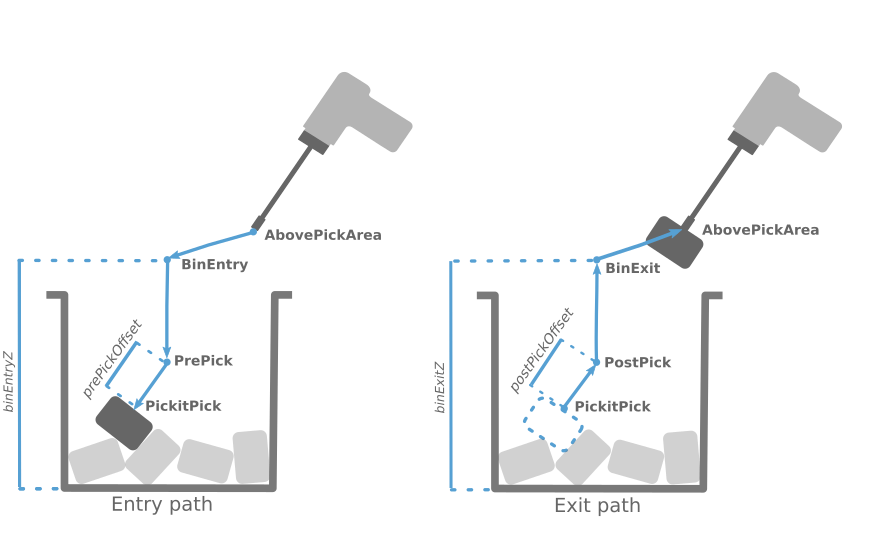
Add grasping/releasing logic
At the F_Pick and Drop points, grasping and releasing logic needs to be added, respectively.
Collision recovery
In KUKA, collision detection is used to monitor the axis torques. If one of these exceed a limit value, the robot stops and operator intervention is needed.
The general preconditions for collision detection are:
$IMPROVED_COLLMON == TRUE
.$ADAP_ACC ≠ #NONE
.The load data are correct.
Collision detection is necessary in bin picking applications to protect the robot and the picking tool.
However, to avoid production downtime, it is recommended to use a collision recovery strategy in the robot program during the Pick()
subroutine.
This way, if the robot collides with an object during picking, the robot does not raise an error requiring operator intervention.
Instead, the robot goes back to the detection point and tries to pick another part.
Collision recovery is implemented in this program.
First, a set of torque sensitivities need to be defined in the respective .DAT
file using the variables iSearchSensitivity[1], iSearchSensitivity[2], ... ,iSearchSensitivity[6]
.
These sensitivities should be lower than the torque limits defined in the enabled collision detection settings.
When one of these limits is crossed during the Pick()
subroutine, a flag CYCFLAG[255]
is raised, which stops the robot motion and calls the RetreatWhenFound()
subroutine.
This will prevent the axis torques from reaching higher values that trigger a robot stop.
When the RetreatWhenFound()
subroutine is executed, the application can be resumed by taking another detection and trying to pick again.
Execute the picking program
Attention
Before running the robot program for the first time, make sure that:
There exists a valid robot-camera calibration.
The Tool Center Point (TCP) has been correctly specified.
The robot speed is set to a low value, so unexpected behavior can be identified early enough to prevent the robot from colliding with people or the environment.
Pickit is in robot mode, which is enabled in the Pickit web interface.
Now you can run the program. Happy picking!